Blog
All Blog Posts | Next Post | Previous Post
Next Generation Data Grid for Delphi: Adding, Formatting & Converting Data
Tuesday, October 1, 2024
Intro
If youre developing in Delphi and looking for a powerful, flexible, and highly customizable data grid solution, then TMS FNC Data Grid is the perfect choice. In this blog, we continue on our journey with the TMS FNC Data Grid. When working with data grids, effectively managing data is essential for creating user-friendly interfaces. Whether you're building dashboards, data entry forms, or reporting tools, TTMSFNCDataGrid makes it simple to add, format, and convert various types of data. In this post, we'll explore how to add data to your grid, format it for better presentation, and convert it between different types all based on the TValue type that powers the grids Cells property.
What is TMS FNC Data Grid?
To have a better understanding on what TMS FNC Data Grid is and has to offer, please read through this blog first.
Understanding TValue and the Cells Property
At the heart of TTMSFNCDataGrid
's data handling is the TValue
type. This flexible type allows you to store different kinds of data (e.g., strings, integers, floating-point numbers, booleans, and TDateTime
) in the grid cells. The grid's Cells
property utilizes TValue
to hold this variety of data seamlessly, making it extremely versatile for any data structure.
Heres a basic example of how to add different types of data to the grid:
Grid.Cells[0, 1] := 1; // Integer Grid.Cells[1, 1] := 'John Doe'; // String Grid.Cells[2, 1] := 'USA'; // String
In this example, we added an integer to the first column, a string in the second column, and another string in the third column. The Cells
property automatically interprets and stores these different data types using TValue
.
Since TValue
supports multiple data types, you can also store floating-point numbers and date/time values:
Grid.Cells[0, 1] := 1234.56; // Floating-point number Grid.Cells[1, 1] := Now; // Current date and time (TDateTime) Grid.Cells[2, 1] := 'USA'; // String
TValue
, TTMSFNCDataGrid
provides great flexibility in handling various data types without needing explicit type conversions, simplifying data management in your grid.// Set the number of rows and columns Grid.RowCount := 2; // 2 rows for more data Grid.ColumnCount := 5; // 5 columns for different data types // Add column headers Grid.Cells[0, 0] := 'Name'; Grid.Cells[1, 0] := 'Join Date'; Grid.Cells[2, 0] := 'Status'; Grid.Cells[3, 0] := 'Years of Experience'; Grid.Cells[4, 0] := 'Salary'; Grid.Cells[0, 1] := 'Alice Johnson'; // Name (string) Grid.Cells[1, 1] := EncodeDate(2022, 5, 22); // Join Date (TDateTime) Grid.Cells[2, 1] := True; // Status (Boolean) Grid.Cells[3, 1] := 5; // Years of Experience (Integer) Grid.Cells[4, 1] := 75000.50; // Salary (Float) Grid.AutoSizeGrid();
TValue
.
Formatting Data in the Grid
TTMSFNCDataGrid
provides powerful formatting options, especially at the column level. This ensures that all cells within a column follow a consistent format.Formatting
property. For example, if youre working with date values, you can format them to display in a specific format, such as yyyy/mm/dd
:Grid.Columns[1].Formatting.&Type := gdftDate; // Set type to Date Grid.Columns[1].Formatting.Format := 'yyyy/mm/dd'; // Display date in yyyy/mm/dd format Grid.Columns[1].AddSetting(gcsFormatting);
By applying formatting at the column level, you ensure that all data in that column is displayed consistently, making your grid data easier to read.
Handling Custom Formatting with Events
In addition to column-level formatting, you can customize the formatting of individual cells by handling the OnGetCellFormatting
event. This event allows you to define specific formatting for different types of data as they are rendered in the grid.
For example, lets say we want to format dates, booleans, and numbers differently:
- Join Date: Display as
yyyy/mm/dd
. - Status: Show
Approved
forTrue
andPending
forFalse
. - Years of Experience: Display as integers.
- Salary: Format as currency with a dollar sign and two decimal places.
Heres how you can handle this in the OnGetCellFormatting
event:
procedure TForm1.GridGetCellFormatting(Sender: TObject; ACell: TTMSFNCDataGridCellCoord; AData: TTMSFNCDataGridCellValue; var AFormatting: TTMSFNCDataGridDataFormatting; var AConvertSettings: TFormatSettings; var ACanFormat: Boolean); begin // Skip formatting for the first row (header) if ACell.Row = 0 then Exit; ACanFormat := True; // Column 1: Format as date if ACell.Column = 1 then begin AFormatting.Format := 'yyyy/mm/dd'; // Custom date format AFormatting.&Type := gdftDate; // Set type to Date end; // Column 2: Format as boolean with custom text if ACell.Column = 2 then begin AFormatting.&Type := gdftBoolean; AFormatting.BooleanTrueText := 'Approved'; AFormatting.BooleanFalseText := 'Pending'; end; // Column 3: Format as integer if ACell.Column = 3 then begin AFormatting.Format := '%g'; // Format integers AFormatting.&Type := gdftNumber; // Set type to Number end; // Column 4: Format salary as currency if ACell.Column = 4 then begin AFormatting.Format := '$#,##0.00'; // Currency format AFormatting.&Type := gdftFloat; // Set type to Float end; end;
procedure TForm1.PopulateGrid; begin // Set the number of rows and columns Grid.RowCount := 5; // 5 rows for more data Grid.ColumnCount := 5; // 5 columns for different data types // Add column headers Grid.Cells[0, 0] := 'Name'; Grid.Cells[1, 0] := 'Join Date'; Grid.Cells[2, 0] := 'Status'; Grid.Cells[3, 0] := 'Years of Experience'; Grid.Cells[4, 0] := 'Salary'; // Add sample data to the grid Grid.Cells[0, 1] := 'Jane Smith'; Grid.Cells[1, 1] := EncodeDate(2022, 5, 22); // Example join date Grid.Cells[2, 1] := False; // Pending Grid.Ints[3, 1] := 5; // Years of experience Grid.Floats[4, 1] := 75000.50; // Salary with decimals Grid.Cells[0, 2] := 'John Doe'; Grid.Cells[1, 2] := EncodeDate(2019, 7, 10); // Example join date Grid.Cells[2, 2] := True; // Approved Grid.Ints[3, 2] := 8; // Years of experience Grid.Floats[4, 2] := 85000.75; // Salary with decimals Grid.Cells[0, 3] := 'Alice Brown'; Grid.Cells[1, 3] := EncodeDate(2021, 11, 3); // Example join date Grid.Cells[2, 3] := False; // Pending Grid.Ints[3, 3] := 3; // Years of experience Grid.Floats[4, 3] := 62000.00; // Salary with no decimals Grid.Cells[0, 4] := 'Bob Johnson'; Grid.Cells[1, 4] := EncodeDate(2018, 9, 15); // Example join date Grid.Cells[2, 4] := True; // Approved Grid.Ints[3, 4] := 12; // Years of experience Grid.Floats[4, 4] := 95000.00; // Salary with no decimals Grid.AutoSizeGrid(); end;
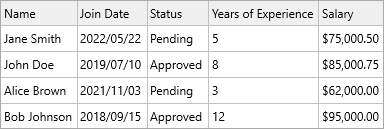
Converting Data
Since TTMSFNCDataGrid
stores its cell data using the versatile TValue
type, its easy to convert between different data types when needed. You can use built-in functions to convert TValue
to specific types, such as integers, floats, booleans, and strings:
- Convert to Integer:
TValue.ToInteger
- Convert to String:
TValue.ToString
- Convert to Float:
TValue.ToFloat
- Convert to Boolean:
TValue.ToBoolean
Example:
var Value: TTMSFNCDataGridCellValue; IntValue: Integer; StrValue: String; begin Value := Grid.Cells[1, 1]; // Fetch cell data as TValue if Value.IsType<Integer> then IntValue := Value.AsInteger; StrValue := Value.AsString; // Convert to string end;
This flexibility allows you to retrieve and manipulate data in the format you need for calculations, validations, or display purposes.
Converting String Data to Dates
Another frequent need is to convert string representations of dates into TDateTime
values. This is common when loading data from external sources, where dates are often stored as strings in various formats.
Example: Converting String Data to TDateTime
Using ConvertSettings
If your grid contains date values stored as strings (e.g., "31-12-2023"
), you can use the ConvertSettings
to transform these strings into TDateTime
values that the grid will display properly:
procedure TForm1.GridGetCellFormatting(Sender: TObject; ACell: TTMSFNCDataGridCellCoord; AData: TTMSFNCDataGridCellValue; var AFormatting: TTMSFNCDataGridDataFormatting; var AConvertSettings: TFormatSettings; var ACanFormat: Boolean); begin // Column 1 is expected to contain date data in string format if ACell.Column = 1 then begin AConvertSettings.ShortDateFormat := 'dd-mm-yyyy'; // Define the input date format AConvertSettings.DateSeparator := '-'; // Specify the date separator AFormatting.Format := 'yyyy/mm/dd'; // Format the output as yyyy/mm/dd AFormatting.&Type := gdftDate; // Indicate the data should be treated as a date ACanFormat := True; // Allow the formatting to proceed end; end;
Here, the ConvertSettings
define how to interpret the input string ("31-12-2023"
) and convert it into a TDateTime
value. The grid will then display the date in the yyyy/mm/dd
format, even though the input was in a different string format.
The TMS FNC Data Grid is a powerful and flexible component for Delphi developers, offering extensive features for displaying, managing, and interacting with data. Whether you're building a desktop, mobile or web application, this grid can handle a wide range of data scenarios while providing a sleek, modern user interface.
In this blog, weve covered how to add, format and convert your data, but its capabilities go far beyond what weve shown here. We encourage you to explore its features to fully unlock its potential in your applications.
In the next blog we'll dive deeper into filtering & sorting, so stay tuned for more advanced tips and tutorials on leveraging this next-generation data grid for your Delphi projects! Happy coding!
Pieter Scheldeman
Related Blog Posts
-
Next Generation Data Grid for Delphi: Getting Started
-
Next Generation Data Grid for Delphi: Adding, Formatting & Converting Data
-
Next Generation Data Grid for Delphi: Filtering & Sorting
-
Next Generation Data Grid for Delphi: Grouping
-
Next Generation Data Grid for Delphi: Webinar Replay Available!
-
Next Generation Data Grid for Delphi: Cell Controls
-
Next Generation Data Grid for Delphi: Master-Detail
-
Next Generation Data Grid for Delphi: Calculations
-
Next Generation Data Grid for Delphi: Import & Export
-
Next Generation Data Grid for Delphi: Template
-
Next Generation Data Grid for Delphi: Filter Row
-
Next Generation Data Grid for C++: Getting Started
-
Freebie Friday: Next Generation Grid Quick Sample Data
-
Next Generation Data Grid for Delphi: Columns Editor
-
Next Generation Data Grid for Delphi: File Drag & Drop
-
Next Generation Data Grid for Delphi: Visual Grouping
-
Next Generation Data Grid for Delphi: FMX Linux Support

This blog post has not received any comments yet.
All Blog Posts | Next Post | Previous Post