Blog
All Blog Posts | Next Post | Previous Post
TMS WEB Core tips & tricks : Small sample with lots to learn about its versatility
Saturday, October 24, 2020
In this article, we start from a TWebResponsiveGrid and use it to demonstrate various tricks that apply throughout the TMS WEB Core framework but are here in combination with the TWebResponsiveGrid perfect to show. The purpose of the article is to show how well the web HTML/CSS world blends with the Delphi developers components world and this in different & flexible ways. We will show you a couple of techniques to customize TWebResponsiveGrid items and make these interactive.TWebResponsiveGrid intro
TWebResponsiveGrid is a responsive grid control. This means that it contains a number of items displayed in a 2D grid layout and this layout, i.e. number of columns will be controlled by the device screen size. This number of columns is determined by the setting TWebResponsiveGrid.Options.ItemMinWidth. This sets the minimum width of items in pixels. So, the grid will automatically show as much columns as allowed to still have this minimum width of items respected. And this also takes the TWebResponsiveGrid.Options.ItemGap in account that specifies the gap between items also in number of pixels. Other than this, there is not much to do. When the TWebResponsiveGrid is resized or displayed on a big or small screen, it will use these settings to automatically render the right number of columns for items. To see this directly live, you can also visit this online demoAn item can be displayed in normal state, hover state and selected state. Therefore
TWebResponsiveGrid.Options has settings for normal state, selected state and hover state colors. In addition or as alternative to setting colors (and other settings) for items, CSS can be used. TWebResponsiveGrid.Options.ItemClassName is the name of the CSS class that can be assigned to the item (outer HTML element of the item is a DIV).
Beyond simple HTML in items
The items one can add in a TWebResponsiveGrid are of the type TWebResponsiveGridItem and have a property HTML: string to set the HTML the item should contain and the generic Tag property. When the TWebResponsiveGrid.Options.ItemTemplate is set, this is preset for the item HTML when a new item is added. Note that when loading data from JSON or a dataset, the template can hold specifiers (%SPECIFIER%) and this is merged at runtime with hthe JSON attribute or dataset record field.The purpose of this article though is on programmatically filling the items collection. The initial code to fill the TWebResponsiveGrid as such is:
for i := 0 to 20 do begin WebResponsiveGrid1.Items.Add.HTML := 'Grid item '+i.ToString+'
This shows how we have added some text and a button to a responsive grid item. The result is very simple. It does not look nice but the responsive behavior is there. The TWebResponsiveGrid is set to top aligned and when resizing the browser, the items automatically flow in the container.
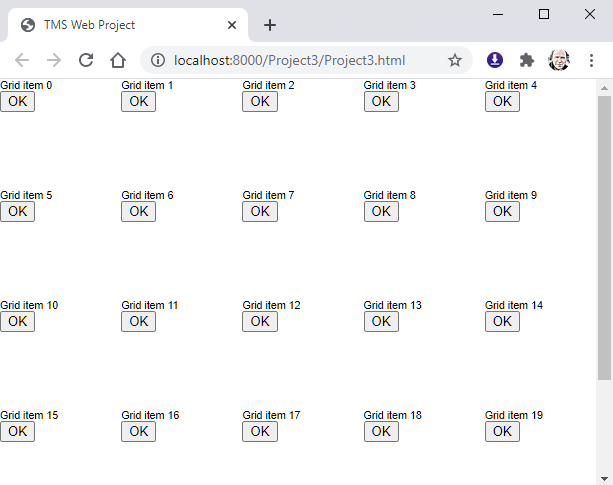
Before diving into making the HTML button react, let's visually enhance the responsive grid items. We do this with some CSS for the TWebResponsiveGrid as well as the TWebResponsiveGridItem. While we could add the CSS style in the HTML file associated with the form or application (which is probably the best place), we wanted to take the opportunity here to introduce the TWebForm.AddCSS() method that allows to dynamically insert CSS at runtime (or remove it later and replace it). Doing so is simple as demonstrated with this code snippet:
AddCSS('respgriditem','.mygriditem { background-color: aliceblue; border-radius: 4px; border: 1px solid darkgray; padding:10px; font-name: Arial; font-size: 12pt;}'); AddCSS('respgrid','.mygrid {padding:10px;}'); WebResponsiveGrid1.ElementClassName := 'mygrid'; WebResponsiveGrid1.Options.ItemClassName := 'mygriditem';
Here you can see a CSS class for the item with some border rounding, padding, font, ...is set and also a CSS based padding added to the TWebResponsiveGrid control itself. The result becomes already a lot nicer:
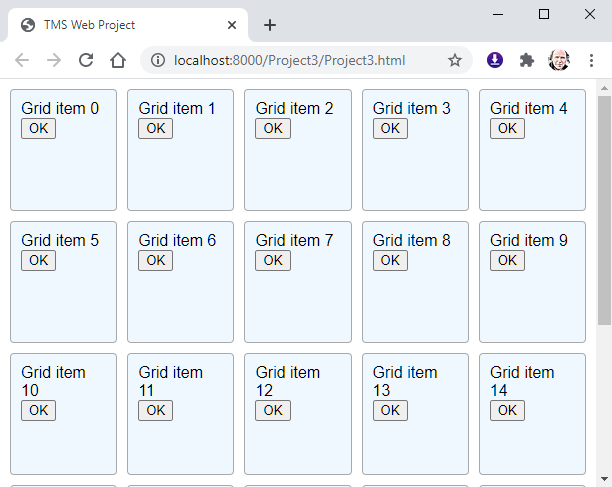
Blending the Object Pascal world with HTML elements
Now comes the more interesting part of this article. It explains two techniques that might not be well-known to bring the RAD component based development world we Delphi developers love so much and the HTML/CSS web world together. First we are going to use a technique to bind a TWebButton control to the HTML in the responsive grid item. By doing this binding, we can do everything we want, including handling events, with the HTML button via the TWebButton class without bothering with the HTML and JavaScript. And doing so is surprisingly simple:TForm3 = class(TWebForm) public { Public declarations } procedure ButtonClick(Sender: TObject); end; procedure TForm3.WebFormCreate(Sender: TObject); var i: integer; btn: TWebButton; begin for i := 0 to 20 do begin WebResponsiveGrid1.Items.Add.HTML := 'Grid item '+i.ToString+'
'; btn := TWebButton.Create('btn'+i.ToString); btn.Tag := i; btn.OnClick := ButtonClick; end; end;
procedure TForm3.ButtonClick(Sender: TObject); begin if (Sender is TWebButton) then WebListbox1.Items.Add('Clicked button ' +(Sender as TWebButton).Tag.ToString); end;
We could use this technique to bind any other type of HTML element to a corresponding TMS WEB Core Pascal class. But, the goal of this article was to introduce lesser known techniques, hence, we will add a checkbox to the item as well with another technique (that could have been used for the button as well).
Clearly, the HTML of the item does not have a HTML INPUT element of the checkbox type, so we are going to add it as a TWebCheckBox. The code to do so is:
for i := 0 to 20 do begin chk := TWebCheckBox.Create(Self); chk.ShowFocus := false; chk.ParentElement := WebResponsiveGrid1.Items[i].ElementHandle; chk.Tag := i; chk.OnClick := ButtonClick; WebResponsiveGrid1.Items[i].ItemObject := chk; end;
chk.ParentElement := WebResponsiveGrid1.Items[i].ElementHandle;
By using a simple trick here associating the created checkbox instance in WebResponsiveGrid1.Items[i].ItemObject, we can later in code manipulate the HTML INPUT CHECKBOX element via the TWebCheckBox class and actually not bother about all the HTML behind. To preset all checkboxes for example, the code is simply:
for i := 0 to 20 do begin (WebResponsiveGrid1.Items[i].ItemObject as TWebCheckBox).Checked := true; end;
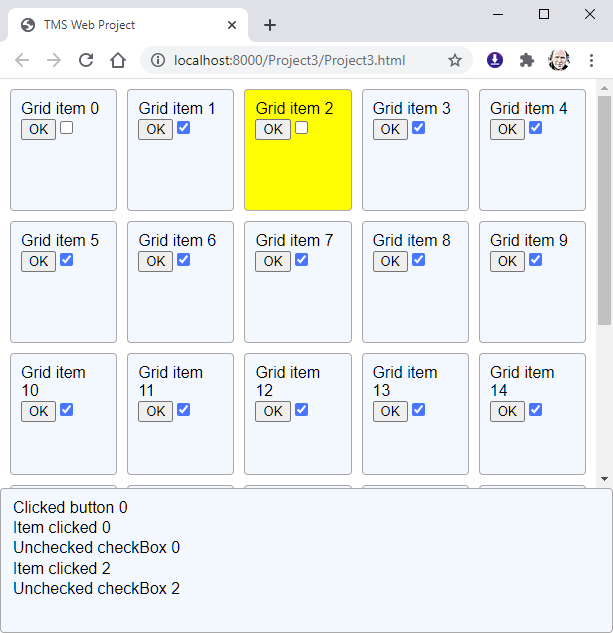
As you can see we have associated the same ButtonClick event handler also to the checkbox OnClick, so we can extend this handler to:
procedure TForm3.ButtonClick(Sender: TObject); begin if (Sender is TWebButton) then WebListbox1.Items.Add('Clicked button ' +(Sender as TWebButton).Tag.ToString); if (Sender is TWebCheckBox) then begin if (Sender as TWebCheckBox).Checked then WebListbox1.Items.Add('Checked checkBox ' +(Sender as TWebCheckBox).Tag.ToString) else WebListbox1.Items.Add('Unchecked checkBox ' +(Sender as TWebCheckBox).Tag.ToString); end; end;
Summary
We hope this small demo you can download here will learn you not only a few handy techniques for working in a flexible way with the TWebResponsiveGrid but as these techniques are generally applicable to all TMS WEB Core controls, will help you to get more out of TMS WEB Core than you already do. Note that everything explained here is also applicable in TMS WEB Core for Visual Studio Code, in fact, the exact same demo project created for Delphi can be directly opened in TMS WEB Core for Visual Studio Code! We look forward to learn how you apply these techniques and what wonderful applications you are creating with TMS WEB Core! If you have suggestions for more in depth looks at TMS WEB Core techniques like this article, let us know and our team will be happy to explore and uncover these in follow-up articles.Bruno Fierens

This blog post has received 5 comments.

Manuel


Bruno Fierens

Manuel

Manuel
All Blog Posts | Next Post | Previous Post
Hennekens Stephan