Frequently Asked Component Specific Questions
Options |
Display all FAQ items |
Displaying items 1 to 1 of 1, page 1 of 1
<< previous next >>



Adding an UIToolBarItem to the toolbar of an UITableView in iCL
The TTMSFMXNativeUITableView has a toolbar property that can be set to True. When adding an item, you can then add it to the TableViewController instead of the NavigationController. Below is the source code that demonstrates this.
unit UDemo; interface uses System.SysUtils, System.Types, System.UITypes, System.Classes, System.Variants, FMX.Types, FMX.Controls, FMX.Forms, FMX.Graphics, FMX.Dialogs, iOSApi.Foundation, FMX.TMSNativeUIBaseControl, FMX.TMSNativeUIView, FMX.TMSNativeUICore, FMX.TMSNativeUIViewController, FMX.TMSNativeUINavigationController, FMX.TMSNativeUIToolBar, MacApi.ObjectiveC, TypInfo, MacApi.ObjcRuntime, FMX.TMSNativeUITableView; type IUIToolBarButtonEventHandler = interface(NSObject) ['{1ACF3DFA-A90B-4F06-ACF1-8A40076BC9ED}'] procedure Click(Sender: Pointer); cdecl; end; TUIToolBarButtonEventHandler = class(TOCLocal) protected function GetObjectiveCClass: PTypeInfo; override; public procedure Click(Sender: Pointer); cdecl; end; TDemoForm = class(TForm) TMSFMXNativeUITableView1: TTMSFMXNativeUITableView; procedure FormCreate(Sender: TObject); private { Private declarations } FEventHandler: TUIToolBarButtonEventHandler; FHideButton: UIBarButtonItem; public { Public declarations } end; var DemoForm: TDemoForm; implementation {$R *.fmx} procedure TDemoForm.FormCreate(Sender: TObject); begin FEventHandler := TUIToolBarButtonEventHandler.Create; FHideButton := TUIBarButtonItem.Wrap(TUIBarButtonItem.Wrap(TUIBarButtonItem.OCClass.alloc).initWithBarButtonSystemItem(integer(siBarButtonSystemItemAdd), FEventHandler.GetObjectID, sel_getUid('Click:'))); TMSFMXNativeUITableView1.Options.ToolBar := True; TMSFMXNativeUITableView1.TableViewController.navigationItem.setRightBarButtonItem(FHideButton); end; { TUIToolBarButtonEventHandler } procedure TUIToolBarButtonEventHandler.Click(Sender: Pointer); begin ShowMessage('clicked'); end; function TUIToolBarButtonEventHandler.GetObjectiveCClass: PTypeInfo; begin Result := TypeInfo(IUIToolBarButtonEventHandler); end; end.
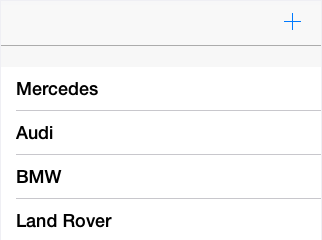