Frequently Asked Component Specific Questions
Options |
Display all FAQ items |
Displaying items 271 to 285 of 888, page 19 of 60
<< previous next >>



TTMSFMXCircularGauge: Subclassing TTMSFMXCircularGauge
When subclassing TTMSFMXCircularGauge, make sure that in the new class, you override the protected method GetDefaultStyleLookupName to ensure your new gauge control uses the base class TTMSFMXCircularGauge default style resource. Additionally for this type of control, you also need to override the GetClassStyleName to return the correct default style. Below is a sample that demonstrates this:
type TFMXWireCircularGauge = class(TTMSFMXCircularGauge) private { Private declarations } protected { Protected declarations } function GetDefaultStyleLookupName: string; override; function GetClassStyleName: String; override; public { Public declarations } procedure ApplyStyle; override; published { Published declarations } end; ... { TFMXWireCircularGauge } procedure TFMXWireCircularGauge.ApplyStyle; begin inherited; end; function TFMXWireCircularGauge.GetClassStyleName: String; begin Result := ClassParent.ClassName + ''style''; Delete(Result, 1, 1); end; function TFMXWireCircularGauge.GetDefaultStyleLookupName: string; begin Result := GetClassStyleName; end;



TTMSFMXEditBtn: How to show a combox with TTMSFMXEditBtn linked to a TFDQuery dataset
You need to link the Text value as you would with a normal TEdit. You can then add a TListBox as dropdown control which is linked to the field of choice. This should work with any control (TListView, TGrid, ...).
Here you can download a sample that demonstrates this.
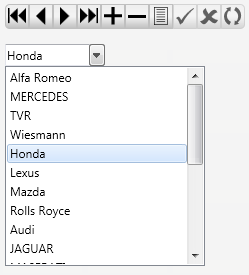



TTMSFMXProgressBar: How to change the color of the progressbar
The TTMSFMXProgressBar inherits from TProgressBar so you can style identical to TProgressBar. You can either change the hindicator or vindicator TRectangle (based on the orientation) in the stylebook, or programmatically In the OnApplyStyleLookup event.
Example:
procedure TForm1.FormCreate(Sender: TObject); begin TMSFMXProgressBar1.Value := 75; end; procedure TForm1.TMSFMXProgressBar1ApplyStyleLookup(Sender: TObject); var r: TRectangle; begin r := TMSFMXProgressBar1.FindStyleResource(''hindicator'') as TRectangle; r.Fill.Color := claRed; r.Fill.Kind := TBrushKind.Solid; end;
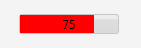



How to add formula support in TAdvSpreadGrid to access DB tables
With this small DB sample mathlib, the concept is demonstrated to add formula support in TAdvSpreadGrid to access DB tables:
type TDBMathLib = class(TMathLib) private { Private declarations } FDataSource: TDataSource; protected { Protected declarations } procedure Notification(AComponent: TComponent; AOperation: TOperation); override; public { Public declarations } function HandlesStrFunction(FuncName:string):Boolean; override; function CalcStrFunction(FuncName:string;Params:TStringList;var ErrType,ErrParam: Integer):string; override; published { Published declarations } property DataSource: TDataSource read FDataSource write FDataSource; end; implementation { TDBMathLib } function TDBMathLib.CalcStrFunction(FuncName: string; Params: TStringList; var ErrType, ErrParam: Integer): string; var s: string; n,e: integer; fld: TField; begin if (FuncName = ''DBV'') then begin if Params.Count <> 2 then begin ErrType := Error_InvalidNrOfParams; Exit; end; if not Assigned(DataSource) then begin ErrType := Error_NoDataSource; Exit; end; if not Assigned(DataSource.DataSet) then begin ErrType := Error_NoDataSet; Exit; end; if not DataSource.DataSet.Active then begin ErrType := Error_NoDataSetActive; Exit; end; s := Params.Strings[0]; // DB FIELD value fld := DataSource.DataSet.FieldByName(s); if not Assigned(fld) then begin ErrType := Error_InvalidValue; ErrParam := 1; end else begin val(Params.Strings[1],n, e); DataSource.DataSet.First; DataSource.DataSet.MoveBy(n); Result := fld.AsString; end; end; end; function TDBMathLib.HandlesStrFunction(FuncName: string): Boolean; begin Result := FuncName = ''DBV''; end; procedure TDBMathLib.Notification(AComponent: TComponent; AOperation: TOperation); begin inherited; if (AOperation = opRemove) and (AComponent = FDataSource) then FDataSource := nil; end;



How to set the background color per item
To set the background color per item, implement OnCustomDrawItem and set the Canvas.Brush.Color from this event.
This example code snippet applies color banding to TTreeList:
procedure TForm1.TreeList1CustomDrawItem(Sender: TCustomTreeView; Node: TTreeNode; State: TCustomDrawState; var DefaultDraw: Boolean); begin if odd(Node.Index) then TreeList1.Canvas.Brush.Color := clInfoBk else TreeList1.Canvas.Brush.Color := clWindow; end;
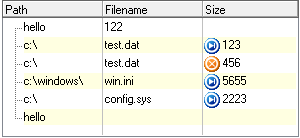



How to copy contents from one TAdvRichEditor instance to another
Copying contents from one TAdvRichEditor instance to another can be done in following way:
var ms: TMemoryStream; begin ms := TMemoryStream.Create; try AdvRichEditor1.SaveToStream(ms); ms.Position := 0; AdvRichEditor2.LoadFromStream(ms); finally ms.Free; end; end;



Programmatically adding items with column values
You use the separator between values of different columns, i.e.:
var tn: TTreeNode; begin tn.Text := ''Column 1 value''+TreeList1.Separator+''Column 2 Value'' .... end;



Showing images in Treelist
To add images to the treelist, please first add a column to the TTreeList where you set TreeList.Columns[index].Image = true
The image displayed in the column for which property Image = true will then be displayed based on the imageindex set for the node.
Note that this way, only one image can be set per node, but with the Columns[index].Image you can choose in what column to set it. If you want to set different images in different columns, I’d recommend to use THTMLTreeList that is basically the same as TTreeList but allows to insert images via HTML tags (<IMG src=”idx:0”>) in text in any column and this way, you could set different images in different columns.



How to add animation when series are added
The TMS Chart for FireMonkey includes a simple animation mode to animate the series from 0 values to the values that are added or vice versa. The AnimationFactor and AnimationFlow properties on Series level determine the speed and mode. There are 2 events that can be used to trace progress: OnAnimateSerieStarted and OnAnimateSerieFinished.
Here you can download a demo that adds animation and triggers it from a button click. Clicking a second time reverts the bars back to zero.
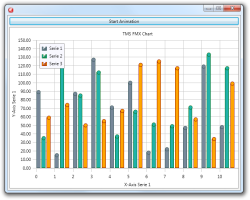



When connecting to FaceBook, I get an error similar to: "The URL entered is not permitted by the application configuration."
Please make sure the “Embedded browser OAuth login” is enabled in your app:
- Go to: https://developers.facebook.com/apps
- Select your app from the Apps dropdown.
- Click on the “Settings” section
- Click on the “Advanced” tab
- Make sure the “Embedded browser OAuth login” switch is set to “YES”
- Save changes



How to verify if TouchID is available on the device
As soon as you add the LocalAuthentication component, you are bound to iOS 8.0. The component already internally checks if authentication is possible before executing it. There is currently no separate verification but if you want to verify if TouchID is available you can use the following code (it requires the FMX.TMSNativeUICore unit)
function TForm1.TouchIDAvailable: Boolean; var ctx: LAContext; error: PPointer; auth: NativeInt; begin result := False; ctx := TLAContext.Wrap(TLAContext.Wrap(TLAContext.OCClass.alloc).init); error := nil; auth := LAPolicyDeviceOwnerAuthenticationWithBiometrics; if ctx.canEvaluatePolicy(auth, error) then Result := True; ctx.release; end;
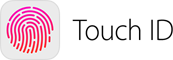



How to update files inside a subfolder under the application folder
If you deployed under C:\Program Files (x86)\ there is no other choice than deploying this as application components (i.e. files should be in CAB and CAB should be listed under appcomps=... in the WebUpdate .INF control file) as typically, your installed app isn’t running with admin permissions to be able to write under the protected \Program Files folder.



How to programmatically set focus to the search box in the grid search footer
You can do this with:
grid.SearchPanel.EditControl.SetFocus;



How to initialize the TAdvGridHeaderList with hidden columns
To allow an easy customization of what columns to show in a grid by the user, an often used user interface is a list holding the available columns and allowing the user to drag & drop from the grid the columns that should be hidden or drag columns that should be displayed from the list to the grid. To achieve this functionality, a component is offered that integrate much of this functionality: TAdvGridHeaderList. The TAdvGridHeaderList is a listbox-style control that holds indexes of available (hidden) columns. By means of drag & drop from the column header to the list and vice versa, it is possible to define what columns to show or not show in the grid.
Here you can download a demo that shows how you can initialize a TAdvGridHeaderList with hidden columns



How to handle the event of changing the combobox from the TColumnComboEditLink component in a TAdvStringGrid
Following code with a TAdvStringGrid and a TColumnComboEditLink on the form demonstrates how you can handle the ComboChange event:
procedure TForm1.AdvStringGrid1GetEditorProp(Sender: TObject; ACol, ARow: Integer; AEditLink: TEditLink); begin ColumnComboEditLink1.Combo.OnChange := ComboChange; end; procedure TForm1.AdvStringGrid1GetEditorType(Sender: TObject; ACol, ARow: Integer; var AEditor: TEditorType); begin AEditor := edCustom; AdvStringGrid1.EditLink :=ColumnComboEditLink1; end; procedure TForm1.ComboChange(Sender: TObject); var cc: TColumnComboBox; begin cc := Sender as TColumnComboBox; advstringgrid1.Cells[0,0] := inttostr(cc.ItemIndex) + ':'+ cc.ComboItems.Items[cc.ItemIndex].Strings[0]; end;
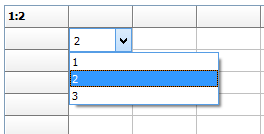