Blog
All Blog Posts | Next Post | Previous Post
Use 100% of TAdvWebBrowser
Monday, November 9, 2020
TAdvWebBrowser/TTMSFNCWebBrowser
TAdvWebBrowser or TTMSFNCWebBrowser are both the same components.The first one is a component that is available in TMS VCL UI Pack and the TTMSFNCWebBrowser is included in TMS FNC Core.
This component can display web pages, HTML and load files such as PDF files.
Both browsers also allow executing scripts and catch the result in a callback.
Get started
You will need to take some additional steps to get started with the TAdvWebBrowser. The component is based on the Microsoft Edge Chromium web browser, so first of all this should be installed. Luckily Edge Chromium is now the default browser that Windows is distributing with the Windows 10 updates.Another important remark is that you can use the normal version of Microsoft Edge and you don't need to intall one of their specific channels.
Another thing that you will need is the WebView2Loader DLL file. There is a 32-bit and 64-bit DLL, the use of one of these depends on the version of the application that you want to build. You can find these files in the 'Edge Support' folder which comes with the installation package.
These need to be placed in the systems folder of your system. In case you are working on a 32-bit system, you will need to put the file in the System32 folder. If you are working on a 64-bit system the file needs to be in the SysWOW64 folder as Delphi is a 32-bit program.
More information on the setup can be found here.
Now you are ready to start working with the TAdvWebBrowser.
TAdvWebBrowser built-in features
To start with the TAdvWebBrowser it can be as easy as placing the component on the form and setting the URL property to the link that you want.If you want to give the user some more control, we've created a demo application, in which you can navigate via a TEdit and have buttons to go forward and backward.
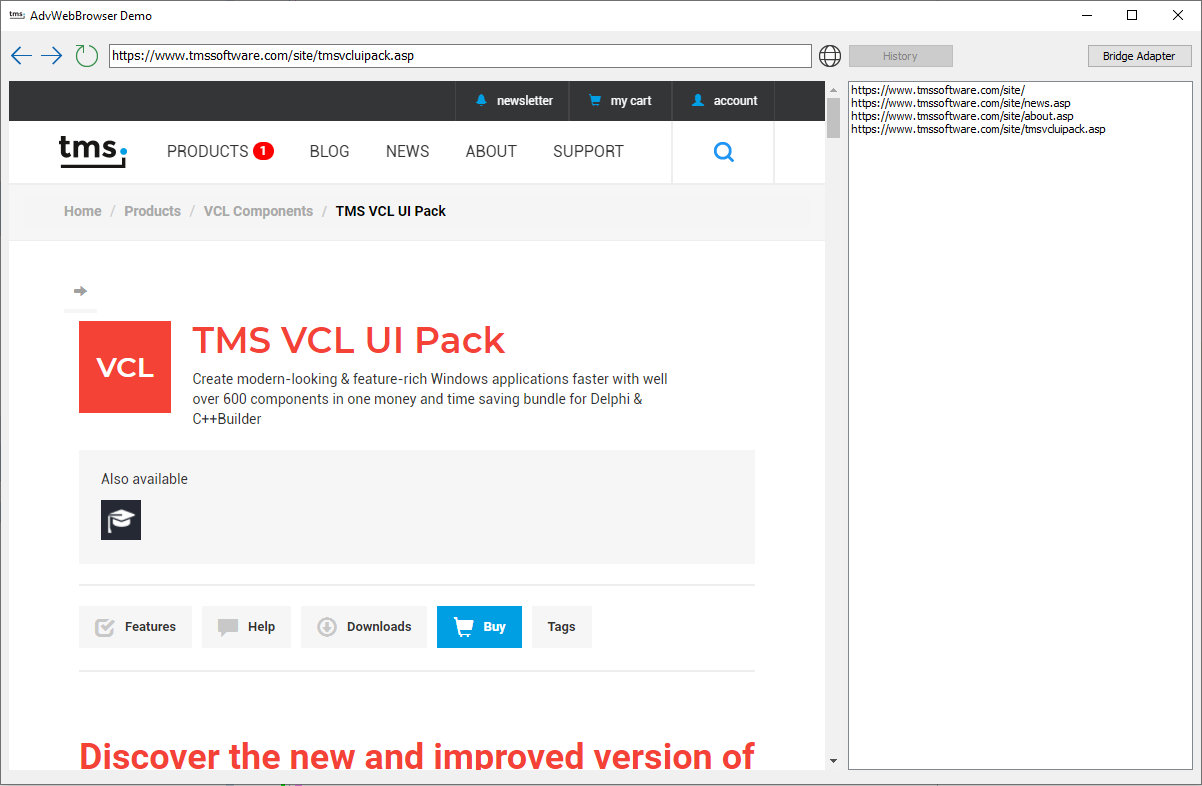
This is easily done with the following code:
AdvWebBrowser.Navigate(AURL); AdvWebBrowser.goBack; AdvWebBrowser.goForward;
In this code sample we will add the URL to a listbox and check if we can go back or forward between the web pages.
procedure TForm.AdvWebBrowserNavigateComplete(Sender: TObject; var Params: TAdvCustomWebBrowserNavigateCompleteParams); begin ListBox1.Items.Add(Params.URL); Back.Enabled := AdvWebBrowser1.CanGoBack; Forward.Enabled := AdvWebBrowser1.CanGoForward; end;
AdvWebBrowser1.LoadHTML(MyHTML);
Go to the next level
You can get much more out of your TAdvWebBrowser with the use of some simple methods.With the ExecuteJavascript method, you can run the JavaScript code that you want in your browser. For example you can set the inner HTML of an object, in this case a paragraph.
AdvWebBrowser.ExecuteJavascript('document.getElementById("myParagraph").innerHTML = "' + s + '";');
AdvWebBrowser.ExecuteJavascript('function GetHTML(){return document.documentElement.innerHTML;} GetHTML();', procedure(const AValue: string) begin memo.Lines.Text:= TJSONObject.ParseJSONValue(AValue).Value;; end );
TAdvWebBrowser also supports bridging between the client and the web browser. You can do this by creating a class for your bridge object.
TMyBridgeObject = class(TInterfacedPersistent, IAdvCustomWebBrowserBridge) private function GetObjectMessage: string; procedure SetObjectMessage(const Value: string); published property ObjectMessage: string read GetObjectMessage write SetObjectMessage; end; procedure TMyBridgeObject.SetObjectMessage(const Value: string); begin //Use the Value parameter. end;
Then you will need to add the bridge to the HTML code.
AHTML := '' + #13 + ' ' + #13 + ' ' + #13 + ' ' + #13 + ' ' + #13 + ' ' + #13 + ' ' + #13 + ''; o := TMyBridgeObject.Create; AdvWebBrowser1.AddBridge(BridgeName, o);
This will give you the following HTML:
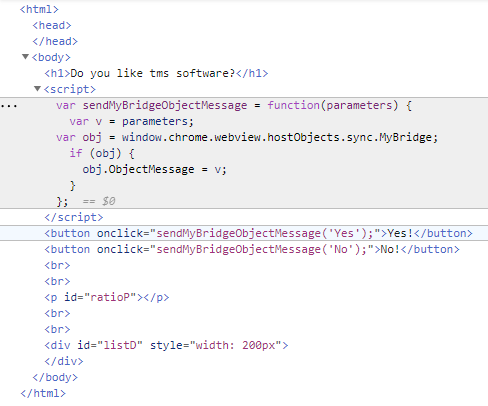
If you want to see the demo in action, I've already made a video on the TAdvWebBrowser demo where I explain how it works.
Gjalt Vanhouwaert

This blog post has received 9 comments.

Ducreux Jean Guy


procedure TForm1.AdvWebBrowser1DocumentComplete(Sender: TObject);
begin
//ready
end;
procedure TForm1.Button1Click(Sender: TObject);
begin
AdvWebBrowser1.URL := ''http://www.tmssoftware.com'';
AdvWebBrowser1.StartDocumentReadyStateThread;
end;
Pieter Scheldeman

Ducreux Jean Guy

Ducreux Jean Guy


Pieter Scheldeman

Nehmer Lizenz

Nehmer Lizenz


Pieter Scheldeman
All Blog Posts | Next Post | Previous Post
E2352 Cannot create instance of abstract class ''TMyBridgeObject''.
class PASCALIMPLEMENTATION TMyBridgeObject : public TInterfacedPersistent, public IAdvCustomWebBrowserBridge
{
typedef TInterfacedPersistent inherited;
private:
String __fastcall GetObjectMessage();
void __fastcall SetObjectMessage( const String Value );
__published:
__property String ObjectMessage = { read = GetObjectMessage, write = SetObjectMessage };
};
Thanks!
mmbuf