Frequently Asked Component Specific Questions
Options |
Display all FAQ items |
Displaying items 376 to 390 of 508, page 26 of 34
<< previous next >>



Remove the context menu at right mouse click
When the inplace editor is active in the TAdvStringGrid, a menu appears when you right click the mouse. This is the default Windows context menu for an edit control. You also see this when dropping a standard VCL TEdit on the form. If you want to remove this, drop a new (empty) TPopupMenu on the form and use the event handler:
procedure TForm4.AdvStringGrid1GetEditorProp(Sender: TObject; ACol, ARow: Integer; AEditLink: TEditLink); begin advstringgrid1.NormalEdit.PopupMenu := PopupMenu1; end;
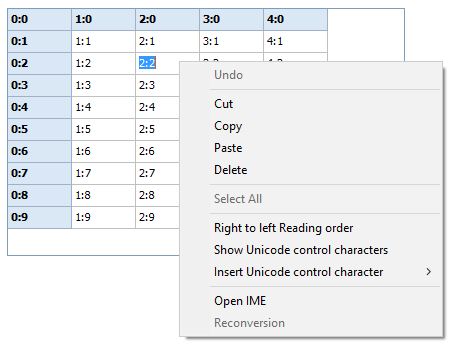



Exporting to PDF
To print the chart to a PDF, you can use following code:
with printer do begin BeginDoc; AdvChartView2.PrintAllPanes(Printer.Canvas,Rect(0, 0, printer.PageWidth, printer.PageHeight)); EndDoc; end;



After installing a new version of the TMS Async and running my application, I get an error similar to : [Dcc32 Fatal Error] VaClasses.pas (25): F2613 Unit 'Graphics' not found.
Make sure that in your project, unit scope names are set to:
Winapi;System.Win;Data.Win;Datasnap.Win;Web.Win;Soap.Win;Xml.Win;Bde;System;Xml;Data;Datasnap;Web;Soap;Vcl;Vcl.Imaging;Vcl.Touch;Vcl.Samples;Vcl.Shell



Putting data in the cloud with myCloudData.net and component TAdvmyCloudData
If we’d want to persist application settings information in the cloud on http://myCloudData.net, this is very simple to do in Delphi.
After connecting with TAdvmyCloudData, we can test for the presence of the table that holds the data to persist, create table & metadata when it doesn’t exist and persist the data.
Code:
var table: TmyCloudDataTable; entity: TmyCloudDataEntity; doins: boolean; begin table := AdvMyCloudData1.TableByName(''APPDATA''); // create table with metadata on the fly if it doesn’t exist for the myCloudData.net account if not Assigned(table) then begin table := AdvMyCloudData1.CreateTable(''APPDATA''); table.MetaData.Add(''WINWIDTH'', ftInteger); table.MetaData.Add(‘WINHEIGHT’,ftInteger); table.MetaData.Add(''WINLEFT'', ftInteger); table.MetaData.Add(''WINTOP'', ftInteger); table.MetaData.Add(''USERNAME'', ftString,50); end; // check for existing entity holding the data table.Query; doins := table.Entities.Count = 0; if doins then entity := table.Entities.Add else entity := table.Entities[0]; // setting data to persist in the entity entity.Value[‘WINWIDTH’] := form.Width; entity.Value[‘WINHEIGHT’] := form.Height; entity.Value[‘WINLEFT’] := form.Left; entity.Value[‘WINTOP’] := form.Top; entity.Value[‘USERNAME’] := GetWindowsUser(); // inserting or updating entity if doins then entity.Insert else entity.Update; end;



Dealing with floating point number precision in TAdvStringGrid
When wanting to keep floating point numbers with high precision in the grid but display with less precision, the OnGetFloatFormat event can be used. In this code snippet, the grid cell value is set with a floating point number with 8 digits but is displayed with only 3 digits precision. Internally, we can keep calculating with values with 8 digits.
Sample:
// Set float value with full precision begin advstringgrid1.FloatFormat := ''%g''; advstringgrid1.Floats[1,1] := 1.23456789; advstringgrid1.Floats[1,2] := 9.87654321; end; // ensure floating point number is only displayed with 3 digits procedure TForm4.AdvStringGrid1GetFloatFormat(Sender: TObject; ACol, ARow: Integer; var IsFloat: Boolean; var FloatFormat: string); begin FloatFormat := ''%.3f''; end; // do calculation at full precision begin advstringgrid1.Floats[1,3] := advstringgrid1.Floats[1,1] + advstringgrid1.Floats[1,2]; end;
1.235
9.877
but the displayed result is
11.111
as the sum calculated is based on full precision.



SelectPosRestricted property
When you want to control that when a user starts a selection within a position (resource) in the Planner, the select-drag will be restricted to the position where the select-drag started, a new property is available:
Planner.SelectPosRestricted: boolean;
Set this to true and even when columns or rows for resources are small and sometimes difficult to keep the mouse in the same resource during long select-drag operations, the selection will remain within the originally selected resource.



Custom X-Axis drawing
procedure TForm1.FormCreate(Sender: TObject); var I: Integer; begin AdvChartView1.BeginUpdate; AdvChartView1.Panes[0].Series[2].Free; AdvChartView1.Panes[0].Series[1].Free; AdvChartView1.Panes[0].Series[0].OnXAxisDrawValue := XAxisDrawVal; AdvChartView1.Panes[0].Series[0].ChartType := ctBar; AdvChartView1.Panes[0].Series[0].XAxis.AutoUnits := False; AdvChartView1.Panes[0].XAxis.AutoSize := False; AdvChartView1.Panes[0].Series[0].AutoRange := arEnabledZeroBased; AdvChartView1.Panes[0].XAxis.Size := 50; for I := 0 to 9 do AdvChartView1.Panes[0].Series[0].AddSinglePoint(Random(100), ''Item '' + inttostr(I)); AdvChartView1.EndUpdate; end; procedure TForm1.XAxisDrawVal(Sender: TObject; Serie: TChartSerie; Canvas: TCanvas; ARect: TRect; ValueIndex, XMarker: integer; Top: Boolean; var defaultdraw: Boolean); var bw, tw: Integer; s: string; begin defaultdraw := False; bw := Serie.GetBarWidth(1) div 2; Canvas.Pen.Color := clBlack; Canvas.Pen.Width := 2; Canvas.MoveTo(XMarker - bw + 1 , ARect.Top); Canvas.LineTo(XMarker - bw + 1, ARect.Top + 10); Canvas.MoveTo(XMarker + bw + 1, ARect.Top); Canvas.LineTo(XMarker + bw + 1, ARect.Top + 10); s := Serie.Points[ValueIndex].LegendValue; tw := Canvas.TextWidth(s); Canvas.TextOut(XMarker - tw div 2, ARect.Top + 12, s); end;
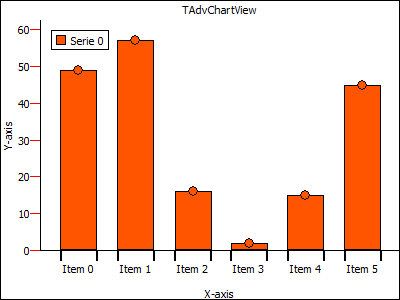



Cloning a TAdvGroupBox at runtime
Cloning a TAdvGroupBox (or any other control) at runtime can be done with following code:
var grp: TAdvGroupBox; begin RegisterClass(TAdvGroupBox); clipboard.SetComponent(advgroupbox1); grp := clipboard.GetComponent(self, self) as TAdvGroupBox; // force position of new clone to 0,0 position grp.Left := 0; grp.Top := 0; end;



How to add a Polyline with symbols to the map
This is a sample of how to add a Polyline with symbols to the map:
var Symbols: TSymbols; Symbol: TSymbol; Path: TPath; begin Path := TPath.Create; Path.Add(48.85, 2.35); Path.Add(52.51, 13.38); Symbols := TSymbols.Create; Symbol := Symbols.Add; Symbol.SymbolType := stForwardClosedArrow; Symbol.OffsetType := dtPixels; Symbol.Offset := 10; Symbol.RepeatValue := 64; Symbol.RepeatType := dtPixels; WebGMaps1.Polylines.Add(false, false, false, Symbols, Path, clBlue, 255, 2, true, 1); end;
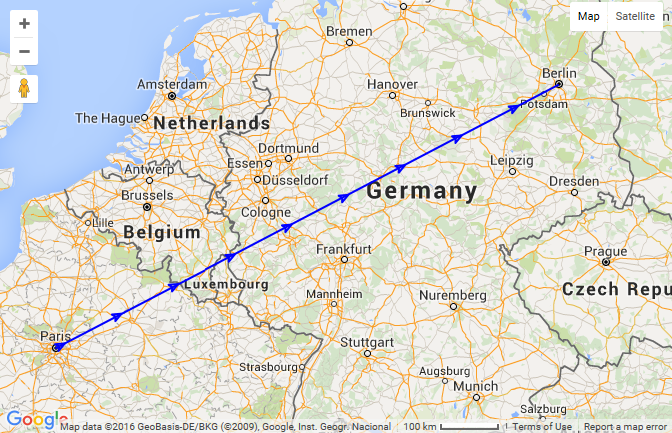



How to export/save an image, displayed in a TAdvSmoothPanel, in its rounded form
The picture is saved as the original picture. Here you can download a sample that demonstrates how you can save the picture as a rounded image .
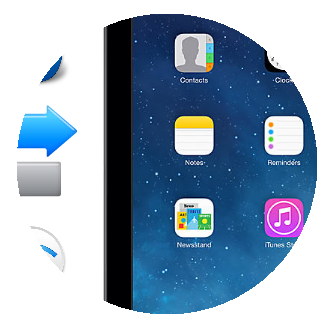



Add array of singles with one function
const val: array[1..12] of Single = (15,23,10,5,3,14,33,11,8,19,9,22); begin TMSFNCChart1.BeginUpdate; TMSFNCChart1.Clear; TMSFNCChart1.AddPointArray(val); TMSFNCChart1.EndUpdate; end;
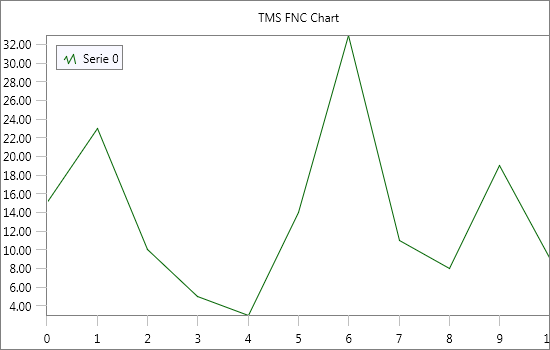



How to center & display all the markers on the map
You can use the Markers.Bounds call to retrieve the bounds coordinates of all markers, these bounds can then be used as a parameter in the MapZoomTo call.
Example:
TMSFMXWebGMaps1.MapZoomTo(TMSFMXWebGMaps1.Markers.Bounds);



How to show a hint when hovering over the column header
You can use the OnGridHint event and via the var parameter hintstr specify the hint for each cell.



Providing credentials to the Google Maps API
There are 2 different methods of providing credentials to the Google Maps API when working with TMS WebGMaps:
1. Regular Google Maps access API Key
https://console.developers.google.com
This key identifies your project to check quotas and access.
At the Google Developers Console, go to "API Manager", "Credentials" to view your existing API Key(s) or create a new one.
You can assign this key to the APIKey property.
2. Google Maps for Work access Client ID and Signature
https://www.google.com/intx/en_uk/work/mapsearth/
https://developers.google.com/maps/premium/overview
These credentials identify your Google Maps for Work project.
After sign up for Google Maps for Work you should receive the required information.
You can assign these to the APIClientID and APISignature properties.



How to create an Excel file with a gradient in a cell, a protected sheet and an autofilter
Excel files can be very complex, and guessing how to for example add a gradient to a cell, or protect a sheet, or add an autofilter can get difficult.
To make it easier, FlexCel provides a very useful tool named APIMate that can "convert" an Excel file into FlexCel code, for either Delphi or C++ Builder.
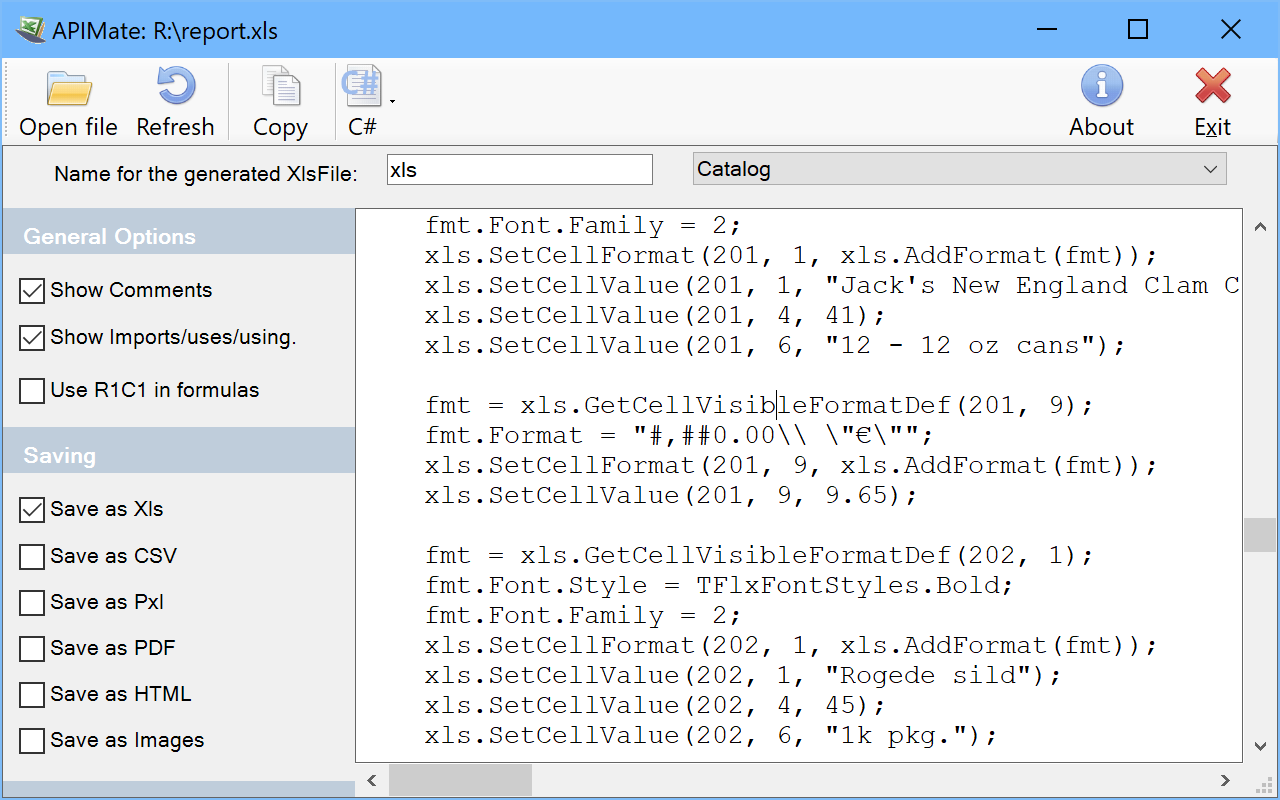
It works like this: You create a file in Excel, and open it in APIMate (without closing Excel). It will tell you the Delphi or C++ code needed to create the file. Then you can make changes in Excel, save the file, and press “Refresh” in APIMate (all without closing Excel). It will tell you the code you need to modify the file from the old state to the new one.
We are now in position to answer the question at the top of this section: How to create a file with a gradient in a cell, a protected sheet and an autofilter? I created a simple file with those things in Excel, and APIMate gives back this code:
procedure CreateFile(const xls: TExcelFile); var fmt: TFlxFormat; GradientStops: TArray<TGradientStop>; SheetProtectionOptions: TSheetProtectionOptions; begin xls.NewFile(1, TExcelFileFormat.v2010); //Create a new Excel file with 3 sheets. //Set the names of the sheets xls.ActiveSheet := 1; xls.ActiveSheet := 1; //Set the sheet we are working in. //Global Workbook Options xls.OptionsCheckCompatibility := false; //Printer Settings xls.PrintXResolution := 600; xls.PrintYResolution := 600; xls.PrintOptions := [TPrintOptions.Orientation]; fmt := xls.GetCellVisibleFormatDef(3, 1); fmt.FillPattern.Pattern := TFlxPatternStyle.Gradient; SetLength(GradientStops, 2); GradientStops[0].Position := 0; GradientStops[0].Color := TExcelColor.FromTheme(TThemeColor.Background1); GradientStops[1].Position := 1; GradientStops[1].Color := TExcelColor.FromTheme(TThemeColor.Accent1); fmt.FillPattern.Gradient := TExcelLinearGradient_Create(GradientStops, 90); xls.SetCellFormat(3, 1, xls.AddFormat(fmt)); //AutoFilter xls.SetAutoFilter(1, 3, 5); //Protection SheetProtectionOptions := TSheetProtectionOptions.Create(false); SheetProtectionOptions.Contents := true; SheetProtectionOptions.Objects := true; SheetProtectionOptions.Scenarios := true; SheetProtectionOptions.SelectLockedCells := true; SheetProtectionOptions.SelectUnlockedCells := true; xls.Protection.SetSheetProtection(''******'', SheetProtectionOptions); end;
APIMate is not the panacea for all the questions, but it helps a lot answering many of those "how do I?" doubts. Remember to use it.