Blog
All Blog Posts | Next Post | Previous Post
TMS X-Cloud Todolist with FNC
Wednesday, June 22, 2016
It's almost three months since we released the first version of the TMS FNC UI Pack, a set of Framework Neutral Components (FNC), and have meanwhile released 2 major updates which include the TTMSFNCTabSet/TTMSFNCPageControl (v1.1), the recently introduced TTMSFNCListBox/TTMSFNCCheckedListBox and significant improvements / new features to the TTMSFNCTreeView such as filtering, sorting, keyboard lookup, clipboard support, ... (v1.2).As already explained in previous blog posts (https://www.tmssoftware.com/site/blog.asp?post=335 and https://tmssoftware.com/site/blog.asp?post=346), the TMS FNC UI Pack is a set of UI controls that can be used from VCL Windows applications, FireMonkey (FMX) Windows, Mac OS-X, iOS, Android applications and LCL framework based Lazarus applications for Windows, Linux, Mac OS-X, ... . The TMS FNC UI Pack contains highly complex & feature-rich components such as grid, planner, rich editor, treeview, toolbars. So, with a single set of controls, you have the freedom of choice to use Delphi, C++Builder or the free Lazarus to create applications for a myriad of operating systems, you have a single learning curve to these components and as demonstrated here, you can use a single source to create apps for multiple targets.
This blog post will cover the TTMSFNCCheckedListBox, which is one of the new components that are added in the latest release (v1.2) of the TMS FNC UI Pack, show how to use myCloudData.net to store data and demonstrate how easy it is to switch between FMX, VCL and LCL with one shared source code. myCloudData is an easy to use & flexible service to make use of structured storage in the cloud from Windows, web, mobile or IoT apps and is offered by tmssoftware.com. myCloudData is OAUTH/JSON REST based and our TMS Cloud Pack includes a component to access the service and thus your data seamlessly.
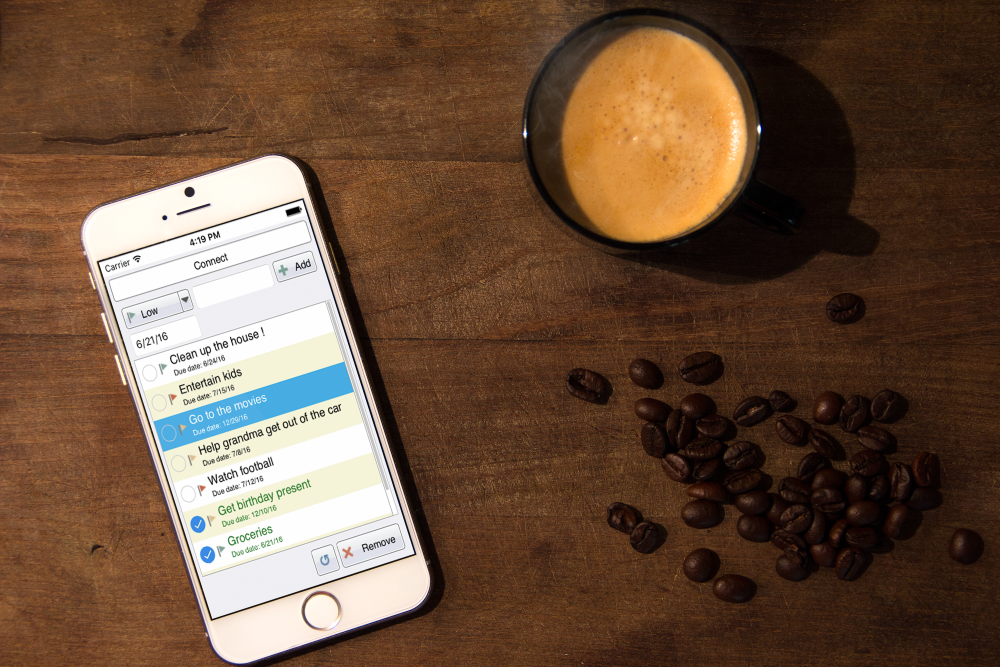
Click image for more screenshots.
A single shared source
As with our TV-guide sample we have created a single shared source file that is used in a FMX, VCL and LCL project. The unit starts by defining the business logic class that will be instantiated in our application main form unit.TTODOListLogic = class private FTable: TMyCloudDataTable; FListBox: TTMSFNCCheckedListBox; FMyCloudDataAccess: TMyCloudDataAccess; FOnConnected: TNotifyEvent; FBitmapContainer: TTMSFNCBitmapContainer; protected procedure DoConnected(Sender: TObject); public destructor Destroy; override; procedure InitListBox(AListBox: TTMSFNCCheckedListBox); procedure InitMyCloudData; procedure Refresh; procedure InitializeTable; procedure AddNewItem(AText: string; ADate: TDateTime; APriority: TPriority); procedure DeleteItem; procedure Connect; procedure DoBeforeDrawItem(Sender: TObject; AGraphics: TTMSFNCGraphics; ARect: TRectF; AItem: TTMSFNCListBoxItem; var AAllow: Boolean; var ADefaultDraw: Boolean); procedure DoItemCheckChanged(Sender: TObject; AItem: TTMSFNCCheckedListBoxItem); procedure DoItemCompare(Sender: TObject; Item1, Item2: TTMSFNCListBoxItem; var ACompareResult: Integer); property OnConnected: TNotifyEvent read FOnConnected write FOnConnected; property BitmapContainer: TTMSFNCBitmapContainer read FBitmapContainer write FBitmapContainer; end;
uses Classes, SysUtils, DB {$IFDEF VCL} ,VCL.TMSFNCListBox, VCL.TMSFNCCheckedListBox, CloudMyCloudData, CloudBase, VCL.TMSFNCUtils, CloudCustomMyCloudData, VCL.TMSFNCGraphics, VCL.Dialogs, VCL.TMSFNCTypes, Types, VCL.TMSFNCBitmapContainer; {$ENDIF} {$IFDEF FMX} ,FMX.TMSFNCListBox, FMX.TMSFNCCheckedListBox, FMX.TMSCloudMyCloudData, FMX.TMSCloudBase, FMX.TMSFNCUtils, FMX.TMSCloudCustomMyCloudData, FMX.TMSFNCGraphics, FMX.TMSFNCTypes, FMX.Dialogs, Types, FMX.TMSFNCBitmapContainer; {$ENDIF} {$IFDEF LCL} ,LCLTMSFNCListBox, LCLTMSFNCCheckedListBox, LCLTMSCloudMyCloudData, LCLTMSCloudBase, LCLTMSFNCUtils, LCLTMSCloudCustomMyCloudData, LCLTMSFNCGraphics, Dialogs, LCLTMSFNCTypes, LCLTMSFNCBitmapContainer; {$ENDIF}
myCloudData
As our todolist is storing its todo items in the cloud we take advantage of our own service, that can virtually store anything we want. The initialization is done programmatically.procedure TTODOListLogic.InitMyCloudData; begin FMyCloudDataAccess := TMyCloudDataAccess.Create(nil); FMyCloudDataAccess.PersistTokens.Location := plIniFile; {$IFDEF FMX} FMyCloudDataAccess.PersistTokens.Key := TTMSFNCUtils.GetDocumentsPath + PthDel + 'myclouddatafmx.ini'; FMyCloudDataAccess.OnConnected := DoConnected; {$ENDIF} {$IFDEF VCL} FMyCloudDataAccess.PersistTokens.Key := TTMSFNCUtils.GetDocumentsPath + PthDel + 'myclouddatavcl.ini'; FMyCloudDataAccess.OnConnected := DoConnected; {$ENDIF} {$IFDEF LCL} FMyCloudDataAccess.PersistTokens.Key := TTMSFNCUtils.GetDocumentsPath + PthDel + 'myclouddatalcl.ini'; FMyCloudDataAccess.OnConnected := @DoConnected; {$ENDIF} FMyCloudDataAccess.PersistTokens.Section := 'tokens'; FMyCloudDataAccess.App.Key := MYCLOUDDATAKEY; FMyCloudDataAccess.App.Secret := MYCLOUDDATASECRET; FMyCloudDataAccess.App.CallBackPort := 8888; FMyCloudDataAccess.App.CallBackURL := 'http://127.0.0.1:8888'; end;
type {$IFDEF VCL} TMyCloudDataAccess = class(TAdvMyCloudData); {$ENDIF} {$IFDEF FMX} TMyCloudDataAccess = class(TTMSFMXCloudMyCloudData); {$ENDIF} {$IFDEF LCL} TMyCloudDataAccess = class(TTMSLCLCloudMyCloudData); {$ENDIF}
procedure TTODOListForm.DoConnected(Sender: TObject); begin Panel1.Enabled := True; Panel2.Enabled := True; TMSFNCToolBarButton2.Enabled := False; TMSFNCCheckedListBox1.Enabled := True; TMSFNCToolBarButton4.Enabled := True; TMSFNCToolBarButton5.Enabled := True; TMSFNCToolBarButton6.Enabled := True; TMSFNCToolBarItemPicker1.Enabled := True; FTODOListLogic.InitializeTable; FTODOListLogic.Refresh; end; procedure TTODOListForm.FormCreate(Sender: TObject); begin FTODOListLogic := TTODOListLogic.Create; FTODOListLogic.InitListBox(TMSFNCCheckedListBox1); FTODOListLogic.InitMyCloudData; {$IFDEF LCL} FTODOListLogic.OnConnected := @DoConnected; {$ELSE} FTODOListLogic.OnConnected := DoConnected; {$ENDIF} TMSFNCCheckedListBox1.BitmapContainer := TMSFNCBitmapContainer1; TMSFNCToolBarItemPicker1.BitmapContainer := TMSFNCBitmapContainer1; TMSFNCToolBarItemPicker1.Bitmaps.Clear; TMSFNCToolBarItemPicker1.Bitmaps.AddBitmapName('low'); TMSFNCToolBarItemPicker1.DisabledBitmaps.Assign(TMSFNCToolBarItemPicker1.Bitmaps); TMSFNCToolBarButton2.DisabledBitmaps.Assign(TMSFNCToolBarButton2.Bitmaps); TMSFNCToolBarButton4.DisabledBitmaps.Assign(TMSFNCToolBarButton4.Bitmaps); TMSFNCToolBarButton5.DisabledBitmaps.Assign(TMSFNCToolBarButton5.Bitmaps); TMSFNCToolBarButton6.DisabledBitmaps.Assign(TMSFNCToolBarButton6.Bitmaps); dt := TMyDateTimePicker.Create(Self); {$IFDEF FMX} dt.Position.X := 5; dt.Position.Y := 40; {$ELSE} dt.Left := 5; dt.Top := 40; {$ENDIF} dt.Date := Now; dt.Parent := Panel1; dt.Width := 105; end; procedure TTODOListForm.FormDestroy(Sender: TObject); begin FTODOListLogic.Free; end; procedure TTODOListForm.TMSFNCCheckedListBox1ItemSelected(Sender: TObject; AItem: TTMSFNCListBoxItem); begin TMSFNCToolBarButton6.Enabled := True; end; procedure TTODOListForm.TMSFNCToolBarButton1Click(Sender: TObject); begin FTODOListLogic.Refresh; end; procedure TTODOListForm.TMSFNCToolBarButton2Click(Sender: TObject); begin FTODOListLogic.Connect; end; procedure TTODOListForm.TMSFNCToolBarButton3Click(Sender: TObject); begin FTODOListLogic.DeleteItem; end; procedure TTODOListForm.TMSFNCToolBarButton4Click(Sender: TObject); begin FTODOListLogic.AddNewItem(Edit1.Text, dt.Date, TPriority(TMSFNCToolBarItemPicker1.SelectedItemIndex)); end; procedure TTODOListForm.TMSFNCToolBarItemPicker1ItemSelected(Sender: TObject; AItemIndex: Integer); begin TMSFNCToolBarItemPicker1.Bitmaps.Clear; TMSFNCToolBarItemPicker1.Bitmaps.AddBitmapName(TMSFNCBitmapContainer1.Items[AItemIndex].Name); TMSFNCToolBarItemPicker1.DisabledBitmaps.Assign(TMSFNCToolBarItemPicker1.Bitmaps); end;
The full source code is available for download
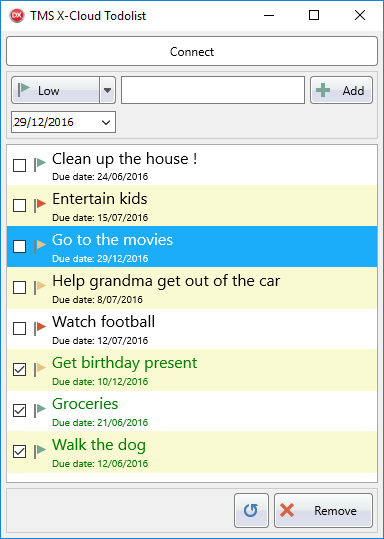
Click image for more screenshots.
Pieter Scheldeman

This blog post has not received any comments yet.
All Blog Posts | Next Post | Previous Post