Blog
All Blog Posts | Next Post | Previous Post
Introducing the new cross-platform .NET SDK for StellarDS.io
Wednesday, March 12, 2025
We're excited to announce the launch of the new StellarDS SDK for .NET! Designed to simplify development and optimize API interactions, this SDK provides strong typing, a structured design, and intuitive features to make integration seamless. Whether you're developing a small-scale application or an enterprise-grade system, the StellarDS .NET SDK ensures that you can leverage StellarDS efficiently with minimal friction.
StellarDS is a fast and seamless backend-as-a-service (BaaS) solution, allowing applications to interact with cloud-based data effortlessly. Through a powerful REST API, StellarDS enables you to retrieve and manipulate data efficiently while handling backend complexities such as storage, security, and scalability. No need to set up servers or implement security protocolsStellarDS takes care of it all!
Key Features of the StellarDS .NET SDK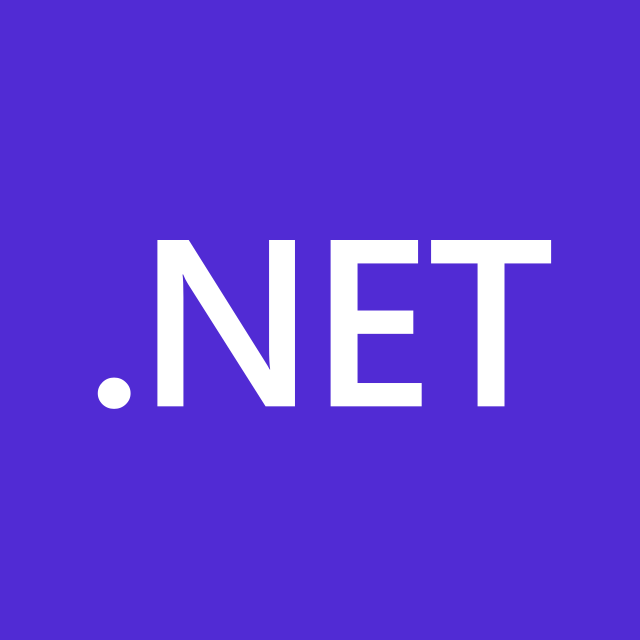
The new .NET SDK introduces several powerful features to enhance API integration:
✅ Strongly Typed Models Leverage C#s type safety to validate API responses and requests at compile time.
✅ Simplified API Calls Perform common operations like data retrieval, updates, and workflow management with minimal boilerplate code.
✅ Asynchronous Programming Take full advantage of async/await for optimal performance in .NET applications.
✅ Comprehensive Documentation Well-documented classes and methods ensure easy implementation and quick onboarding.
✅ Enhanced Developer Experience Minimize runtime errors and improve maintainability with strongly-typed API interactions.
Cross-Platform Compatibility
The StellarDS .NET SDK is designed to work seamlessly across multiple platforms. Whether you're developing a web application in ASP.NET MVC, building interactive UI components in Blazor, creating desktop applications with WPF and WinForms, or targeting mobile and cross-platform development with .NET MAUI, this SDK ensures smooth integration and performance.
Making API Calls with the .NET SDK
Once authenticated, you can start making API requests to interact with StellarDS data. Below are examples of how to fetch and manipulate data using the SDK.
GET Request
To fetch a list of tables from your StellarDS project, use the following code:
using StellarDs.SDK.Api; using StellarDs.SDK.Client; Configuration config = new Configuration(); // Configure API key authorization: Bearer config.AddApiKey("Authorization", "YOUR_API_KEY"); config.AddApiKeyPrefix("Authorization", "Bearer"); var apiInstance = new DataApi(config); var project = "project_example"; // Guid | The project containing the table. var table = 789L; // long | Id of the table containing the records. var offset = 789L; // long? | The offset of the records. (optional) var take = 789L; // long? | The amount of records. (optional) var joinQuery = "joinQuery_example"; // string? | The join queries to apply. (optional) var whereQuery = "whereQuery_example"; // string? | The where queries to apply. (optional) var sortQuery = "sortQuery_example"; // string? | The sort queries to apply. (optional) var distinct = false; // bool? | (optional) (default to false) var select = "select_example"; // string? | (optional) try { // Gets the records for a given table. AbstractObjectQueryResult result = apiInstance.Get(project, table, offset, take, joinQuery, whereQuery, sortQuery, distinct, select); Debug.WriteLine(result); } catch (ApiException e) { Debug.Print("Exception when calling DataApi.Get: " + e.Message); Debug.Print("Status Code: " + e.ErrorCode); Debug.Print(e.StackTrace); }
POST Request
Adding a record to a table is straightforward with the SDK:
using StellarDs.SDK.Api; using StellarDs.SDK.Client; Configuration config = new Configuration(); // Configure API key authorization: Bearer config.AddApiKey("Authorization", "YOUR_API_KEY"); config.AddApiKeyPrefix("Authorization", "Bearer"); var apiInstance = new DataApi(config); var project = "project_example"; // Guid | The project containing the table. var table = 789L; // long | The table to be added to. var createRecordRequest = new CreateRecordRequest?(); // CreateRecordRequest? | (optional) try { // Adds records to the given table. AbstractObjectQueryResult result = apiInstance.Post(project, table, createRecordRequest); Debug.WriteLine(result); } catch (ApiException e) { Debug.Print("Exception when calling DataApi.Post: " + e.Message); Debug.Print("Status Code: " + e.ErrorCode); Debug.Print(e.StackTrace); }
Installing the StellarDS .NET SDK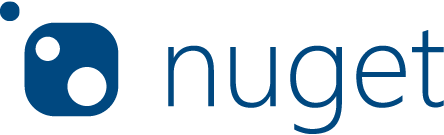
You can install the StellarDS .NET SDK easily using NuGet. Open the Package Manager Console in Visual Studio and run the following command:
Install-Package Stellards.SDK
Alternatively, you can install it using the .NET CLI:
dotnet add package Stellards.SDK
Once installed, you can start integrating StellarDS into your application with minimal setup.
Get Started Today
The StellarDS .NET SDK is available now, complete with sample code and detailed documentation to help you get started quickly. Check out our demo application, explore the SDKs capabilities, and start building powerful applications with StellarDS today.
📥 Download the SDK, check out the repository on GitHub or Read the Documentation to get started now!
Follow us now!
Show us support and follow us on our social media to always be up to date about stellards.io
Bradley Velghe

This blog post has not received any comments yet.
All Blog Posts | Next Post | Previous Post