Tips and Frequently Asked Questions

Toggle between TMS Office hints and regular hints

How to use TAdvOfficeHint for controls that do not have an OfficeHint property

Changing font and font size in TAdvMenuOfficeStyler does not work

Programmatically make toolbar floating and dock toolbar

Using a toolbar customizer

Inserting, updating a menu item at runtime

Changing the display time of TAdvOfficeHint

Programmatically adding items to the application menu

Programmatically adding page, toolbar and button to the ribbon

Providing an Office 2007 type hint for any control

Starting with TMS ToolBars & Menus to create Office 2003 style applications

Using the TMS TAdvMenus with the TAdvToolBar

Starting with TMS ToolBars & Menus to create Office 2007 style applications

Handling shortcuts

Upgrading from TAdvToolBar v1.x

Using menu items with Notes
Pricing
Single Developer License
Small Team License
Site License
TMS VCL UI Pack
€ 375
€
150
yearly renewal
license for 1 developer
Includes
check
Full source code
check
Access to the TMS Support Center
check
Free updates and new releases
MOST POPULAR
TMS VCL Subscription
€ 895
€
450
yearly renewal
license for 1 developer
Includes
check
Full source code
check
Access to the TMS Support Center
check
Free updates and new releases
check
TMS VCL UI Pack
check
All TMS VCL products
more_horiz
Discover more
BEST VALUE
TMS ALL-ACCESS
€ 1,795
€
575
yearly renewal
license for 1 developer
Includes
check
Full source code
check
Access to the TMS Support Center
check
Free updates and new releases
check
TMS VCL UI Pack
check
All TMS VCL products
check
All TMS products
more_horiz
Discover more
All prices excl. VAT. Renewal price is subject to change and only valid up to 30 days after license has expired. After renewal period a discount price is offered to renew the license.
Free Trial
Start a free TMS VCL UI Pack evaluation today!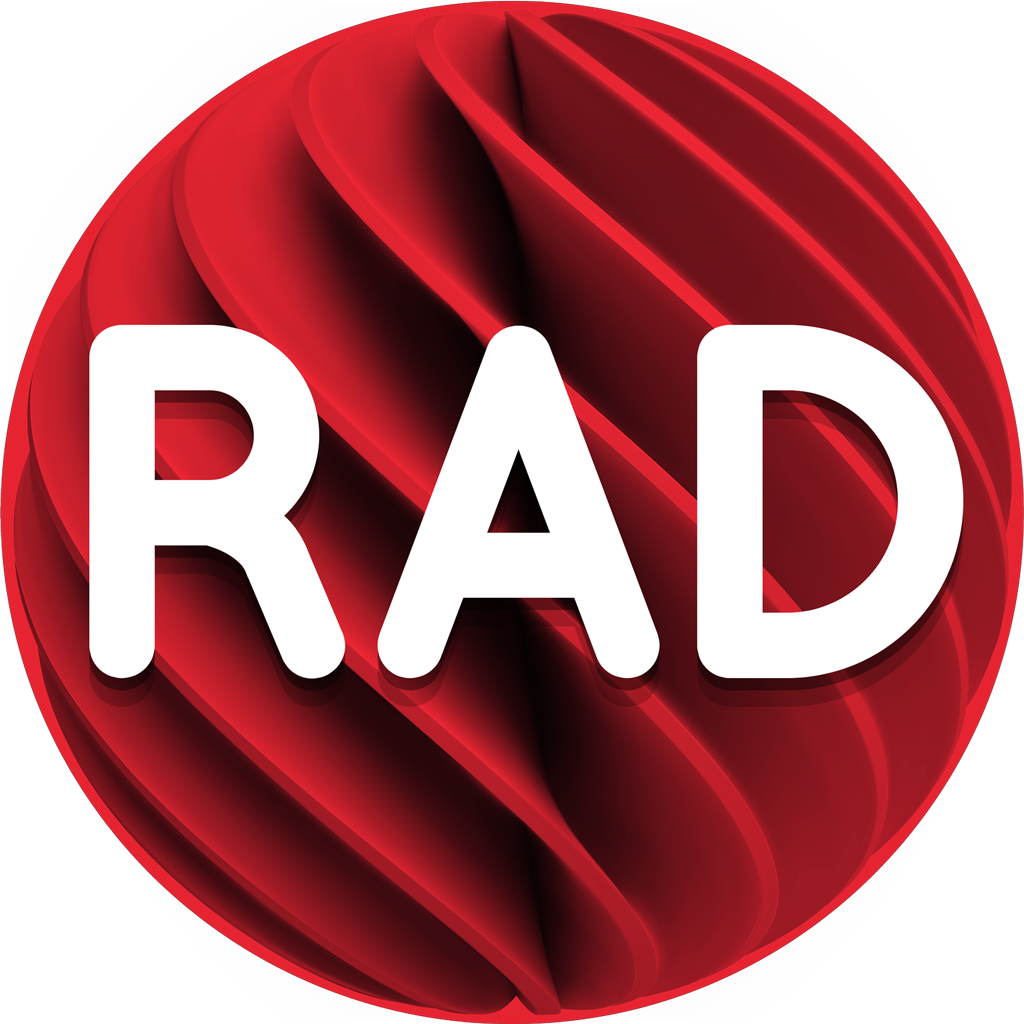
Note that the TMS VCL UI Pack replaces the TMS Component Pack. Both products can't be installed simultaneously. Therefore TMS Component Pack must first be uninstalled before installing the TMS VCL UI Pack