Tips and Frequently Asked Questions

FullWordAtXY() and TokenAtXY() methods

How to set the cursor position at the beginning of a line when clicking in the TAdvMemo

How to synchronise the vertical scrolling of 2 TAdvMemo Objects

How to control the vertical scroll position

How to use bookmarks

How to insert text in a new line

How to create own Syntax Highlighters with customer specified keywords

How to show a breakpoint indicator for a line

How to use Autocompletion in TAdvMemo

How to check if a TAdvMemo is empty

How to add Emoticons programatically

Using the design time editor at runtime

Programmatically find and select text in the memo

The font keeps resetting to FixedSys when trying to change it
Pricing
Single Developer License
Small Team License
Site License
TMS VCL UI Pack
€ 375
€
150
yearly renewal
license for 1 developer
Includes
check
Full source code
check
Access to the TMS Support Center
check
Free updates and new releases
MOST POPULAR
TMS VCL Subscription
€ 895
€
450
yearly renewal
license for 1 developer
Includes
check
Full source code
check
Access to the TMS Support Center
check
Free updates and new releases
check
TMS VCL UI Pack
check
All TMS VCL products
more_horiz
Discover more
BEST VALUE
TMS ALL-ACCESS
€ 1,795
€
575
yearly renewal
license for 1 developer
Includes
check
Full source code
check
Access to the TMS Support Center
check
Free updates and new releases
check
TMS VCL UI Pack
check
All TMS VCL products
check
All TMS products
more_horiz
Discover more
All prices excl. VAT. Renewal price is subject to change and only valid up to 30 days after license has expired. After renewal period a discount price is offered to renew the license.
Free Trial
Start a free TMS VCL UI Pack evaluation today!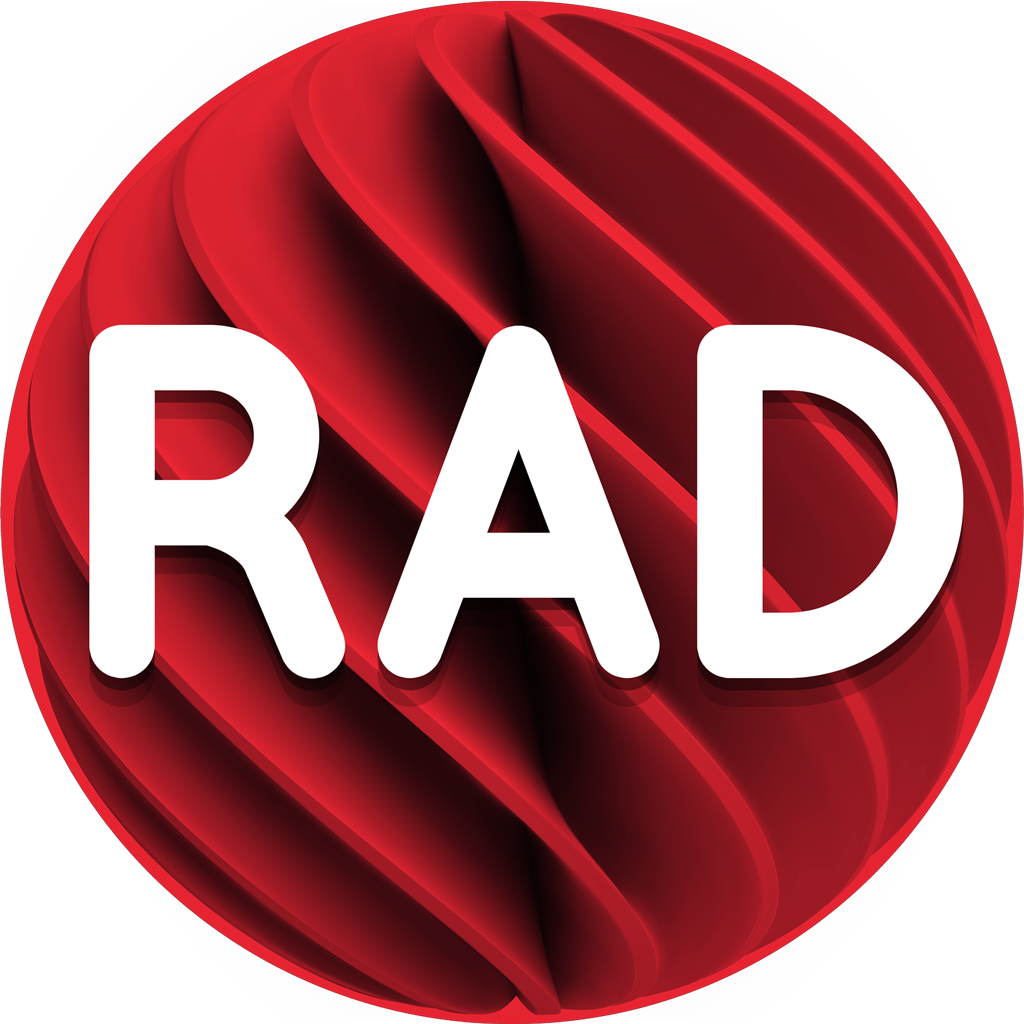
Note that the TMS VCL UI Pack replaces the TMS Component Pack. Both products can't be installed simultaneously. Therefore TMS Component Pack must first be uninstalled before installing the TMS VCL UI Pack